ref: https://medium.com/cubemail88/c-dev-and-debug-with-docker-containers-c89b851a4b69
C++ dev and debug with Docker containers
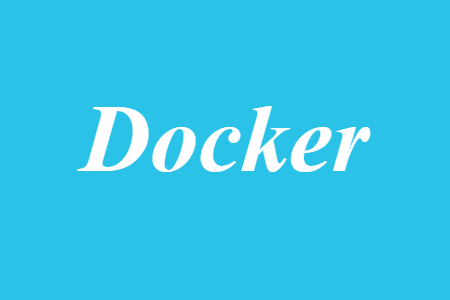
Recently we were testing our c program in ubuntu, but we didnt have enough machine and the priority to build the VM, so my colleague and i decided to make a Dockerfile with c++ setting and run in the container
example from the github project (reference to https://github.com/leimao/CPP_Debug_Docker)
Clone the project
git clone https://github.com/LeoLiu1209/CPP_Debug_Docker.git
Create Docker image(already exists)
add some packages like vim, git, valgrind, gdb, cmake, build-essential….
FROM ubuntu:18.04 | |
LABEL maintainer="Lei Mao <dukeleimao@gmail.com>" | |
# Install necessary dependencies | |
RUN apt-get update &&\ | |
apt-get install -y --no-install-recommends \ | |
build-essential \ | |
autoconf \ | |
automake \ | |
libtool \ | |
pkg-config \ | |
apt-transport-https \ | |
ca-certificates \ | |
software-properties-common \ | |
wget \ | |
git \ | |
curl \ | |
gnupg \ | |
zlib1g-dev \ | |
swig \ | |
vim \ | |
gdb \ | |
valgrind \ | |
locales \ | |
locales-all &&\ | |
apt-get clean | |
# Install CMake | |
RUN wget -O - https://apt.kitware.com/keys/kitware-archive-latest.asc 2>/dev/null | apt-key add - &&\ | |
apt-add-repository "deb https://apt.kitware.com/ubuntu/ bionic main" &&\ | |
apt-get update &&\ | |
apt-get install -y cmake | |
ENV LC_ALL en_US.UTF-8 | |
ENV LANG en_US.UTF-8 | |
ENV LANGUAGE en_US.UTF-8 |
build the docker image
- -f: Dockerfile path
- -t: Create a tag TARGET_IMAGE that refers to SOURCE_IMAGE
docker build -f Dockerfile -t cppdebug:0.1 .
Start Docker Container
- -v : volume path, link current local path to docker /mnt directory
docker run -it --rm --cap-add=SYS_PTRACE --security-opt seccomp=unconfined -v $(pwd):/mnt cppdebug:0.1
ps: if you want ot using gdb as debugger in docker, it’s important to set the arguments --cap-add=SYS_PTRACE
and --security-opt seccomp=unconfined
both of them are required for C++ memory profiling and debugging in Docker.
reference:
GDB Done!!!

CMakeList.txt:
Here is my CMakeLists.txt
file that CMake to generate the platform-specific Makefiles for C++ demo programs
cmake_minimum_required(VERSION 3.13.0) | |
project(DEBUG_DEMO VERSION 0.0.1 LANGUAGES CXX) | |
set(CMAKE_CXX_STANDARD 11) | |
# Non-optimized code with debug symbols | |
# This is equivalent to `g++ -g xxx.cpp -o xxx` | |
set(CMAKE_BUILD_TYPE Debug) | |
# Optimized code with debug symbols | |
#set(CMAKE_BUILD_TYPE RelWithDebInfo) | |
# Optimized code for release | |
#set(CMAKE_BUILD_TYPE Release) | |
add_library(header header.cpp) | |
add_executable(logicalError logicalError.cpp) | |
target_link_libraries(logicalError PRIVATE header) | |
add_executable(coreDumped coreDumped.cpp) | |
add_executable(memoryLeak memoryLeak.cpp) |
Let’s do it with cmake
cd mnt # docker file path link to local path
cmake . #Current workspace
make #build the binary
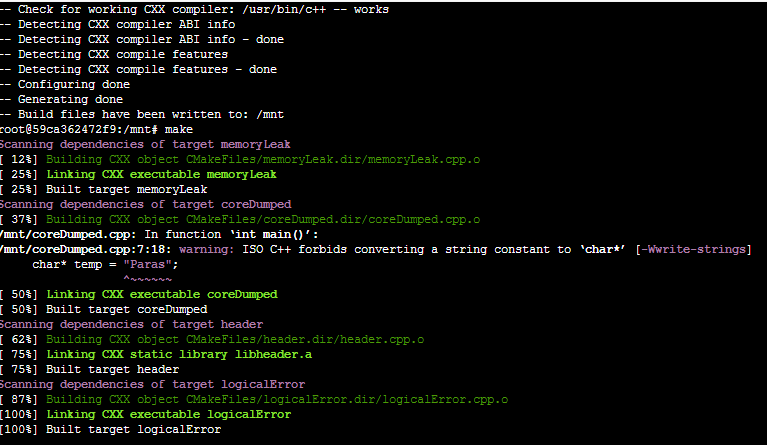
Memory Leak checker -Valgrind
we use Valgrind to check for the memory leak, so this time we add valgrind to makefile then you dont need to type it again and again
Option: --leak-check=yes
do make clean
to clean the binary
cmake_minimum_required(VERSION 3.13.0) | |
project(DEBUG_DEMO VERSION 0.0.1 LANGUAGES CXX) | |
set(CMAKE_CXX_STANDARD 11) | |
# Non-optimized code with debug symbols | |
# This is equivalent to `g++ -g xxx.cpp -o xxx` | |
set(CMAKE_BUILD_TYPE Debug) | |
# Optimized code with debug symbols | |
#set(CMAKE_BUILD_TYPE RelWithDebInfo) | |
# Optimized code for release | |
# set(CMAKE_BUILD_TYPE Release) | |
add_library(header header.cpp) | |
add_executable(logicalError logicalError.cpp) | |
target_link_libraries(logicalError PRIVATE header) | |
add_executable(coreDumped coreDumped.cpp) | |
add_executable(memoryLeak memoryLeak.cpp) | |
find_program(VALGRIND "valgrind") | |
if(VALGRIND) | |
add_custom_target(valgrind COMMAND "${VALGRIND}" --tool=memcheck --leak-check=yes --show-reachable=yes --num-callers=20 --track-fds=yes $<TARGET_FILE:memoryLeak>) | |
endif() |
cmake again cmake .
then you can using make valgrind
now!!!!
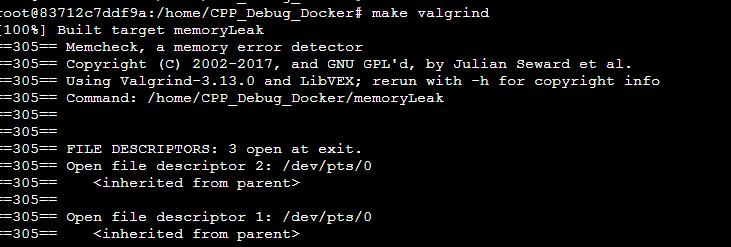
I have to say that Docker really save our life!!!!!!!!!!!
short note for u and me ❤️
No comments:
Post a Comment